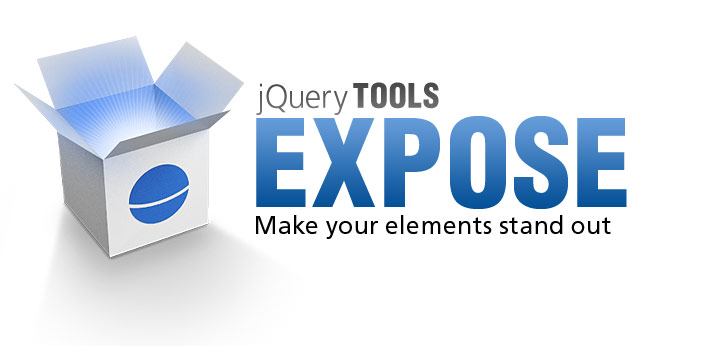
Expose
Start entering text on the input fields below and you'll see the masking effect:
The story
Expose is a JavaScript tool that exposes selected HTML elements on the page so that the surrounding elements will gradually fade out. The exposing effect was first introduced in the overlay tool. Usually the effect is an integral part of the program and cannot be used separately. This tool takes the idea of exposing a little further. It is a separate tool that can be used as a general purpose masking utility. You can use it for overlays, forms, images, videos or Flash objects. You can use CSS to tweak the look of the mask.
Usage
Here are some most common ways of using the mask:
// place a white mask over the page
$(document).mask();
// place a custom colored mask over the page
$(document).mask("#789");
// place a non-closable mask
$(document).mask{ closeOnEsc: false, closeOnClick: false });
// place a mask but let selected elements show through (expose)
$("div.to_be_exposed").expose();
// close the mask
$.mask.close();
There are two different calls: mask and expose. The mask method is only available for the document object and you cannot use any other selector. The expose() method can take any jQuery selector and all elements returned by the selector are placed on top of the mask.
The mask is loaded immediately after the expose or mask call. You can supply a different configuration on each call and the latest call is remembered. A subsequent expose call for example will use the previously used configuration if no arguments are given.
You can also use an existing element as a mask. By default the tool uses an element whose id is exposeMask and if it does not exist already exist then it will be created automatically.
Demos
We believe that the best way to learn is through demos. The following demos are fully documented and a standalone page is provided to get mask working on your site. It's really important to study the first demo "Minimal setup for mask" because it teaches you the basics of using the library.
Expose and Flowplayer demos
These demos show how to use a video player together with the mask tool:
- Exposing video upon mouseover
- Exposing videos
- Custom fullscreen action with overlay and mask
- Email and embed features with overlay and mask
These graphics are being used as the mask's background image. JPG versions are smaller, but they have a fixed background color. PNG images are larger, but you can use any background color in them. Here is a cool example where the mask's background image has been changed.
expose Graphics
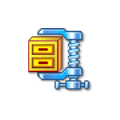
You can use our graphics as the basis for your design. You can freely change the design as you see fit. Click the image on the right to download a zip file. Before using the graphics, you should consult the User's Guide on how graphics can be used when designing the look and feel of the tools.
Here are a few examples of what is included in the zip file:
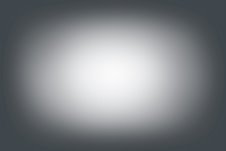
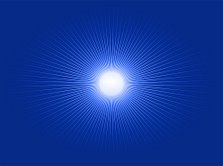
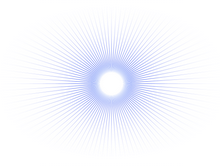
Configuration
Here is a full list of available configuration options:
Here is an example of an onBeforeLoad callback function given in configuration. Inside any callback function the this variable is a pointer to the mask scripting API.
$("#mydiv").expose({
// before masking begins ...
onBeforeLoad: function(event) {
// grow the exposed elements smoothly by 100 pixels
this.getExposed().animate({width: '+=100'});
}
});
Note: unlike other jQuery Tools you can supply callbacks only from the configuration and you can assign only one callback for the same event.
Scripting API
Unlike other jQuery Tools in this library the mask is a singleton. Only one mask instance can exist at any given time. This singleton can be accessed directly with $.mask or via the this variable inside callback functions.
Method listing
method | return value | description/example |
---|---|---|
close() | API |
Closes the mask. |
fit() | API |
Forces the mask to fill the whole document. This is usually not needed since the tool itself does this. You'll need this on occasions when the mask is loaded and your document has grown in size in the meantime. |
isLoaded(fully) | boolean |
Returns true if mask is loaded. Since 1.2.4 you can supply a boolean argument that makes sure that the function returns true only if the mask is fully visible in it's final position and opacity. |
getMask() | jQuery |
Returns the mask as a jQuery object. You can use jQuery methods such as css or animate to modify it. |
getExposed() | jQuery |
Returns exposed elements (if any) as a jQuery object. |
getConf() | Object |
Returns the masking configuration. |