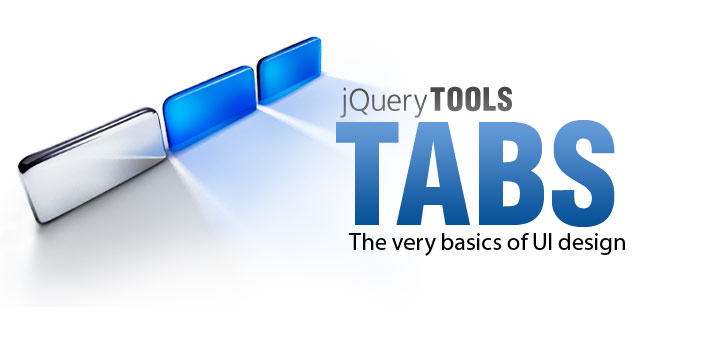
Tab tool basics
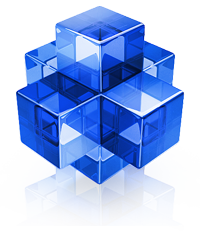
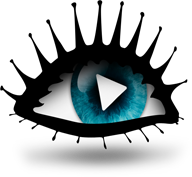
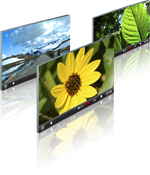
Simple
The above tabs are initialized with this JavaScript one-liner $(".tabs").tabs(".panes > div");. The rest is CSS coding. The core of the tabs is plain and simple. There are no redundant features.
Fading, sliding, ajaxed, horizontal ...
The logic in tabs is simple. You click on something and it does something elsewhere. This tool has an effect framework that generalizes this logic making it possible to do wildly different things such as accordions or crossfading tabs.
Respects browsers' back button
You can enable history support for tabs so that end users can navigate between tabs using their browser's back and forward buttons. Just like everything else this works in all browsers.
File size: 0.9 Kb
And you'll have all the features and configuration options you'll possibly need, such as effects and a plugin framework, scripting API and an event model. A smaller codebase is easier to control and results in snappier behaviour. Without gzipping the size is 2.9 Kb.
Quick Start
Here is the minimal HTML code to get tabs working:
<!-- the tabs -->
<ul class="tabs">
<li><a href="#">Tab 1</a></li>
<li><a href="#">Tab 2</a></li>
<li><a href="#">Tab 3</a></li>
</ul>
<!-- tab "panes" -->
<div class="panes">
<div>pane 1 content</div>
<div>pane 2 content</div>
<div>pane 3 content</div>
</div>
JavaScript setup
This JavaScript snippet activates those tabs:
// setup ul.tabs to work as tabs for each div directly under div.panes
$("ul.tabs").tabs("div.panes > div");
The rest is CSS coding. Look at the standalone version and its source code to get things working on your site.
Since v1.2.3 another $("ul.tabs").tabs() call would destroy the existing instance and will install a completely new tabs instance.
Demos
It's important to study the first demo, "Minimal setup for tabs", because it teaches you the basics of using the library.
- Minimal setup for tabs
- Naming the tabs
- 4 different skins with CSS
- Using mouseover to switch tabs
- Making wizards with the tabs
- Making accordions with tabs
- Customizing the accordion effect
- Horizontal accordion using the tabs
- Multiple tabs and accordion instances
- Handling browsers back button
- Loading tab contents with ajax
- AJAXed tabs with history support
- Slideshow plugin for the tabs
tabs Graphics
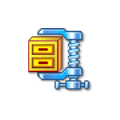
You can use our graphics as the basis for your design. You can freely change the design as you see fit. Click the image on the right to download a zip file. Before using the graphics, you should consult the User's Guide on how graphics can be used when designing the look and feel of the tools.
Here are a few examples of what is included in the zip file:
These graphics are being used in the following CSS files:
They are using a technique called CSS sprites which is a very important technology in tab design.Configuration
There are many other ways of using this tool than the demos provided above. Here is a generic form for constructing tabs:
$("<tabs_selector>").tabs("<pane_selector>", {
/* tabs configuration goes here */
// first configuration property
event: 'mouseover',
// another property
effect: 'slide',
// ... the rest of the configuration properties
});
tabs_selector
You select one or more root elements for the tabs with the tabs_selector which is a jQuery selector. The root element is a container for individual tabs. By default, the tool searches for a tags inside the root element that will work as individual tabs. This can be changed with the tabs configuration option. The root element can be any HTML element such as div, ul, dl or table.
1st argument: pane_selector
In Tabs terminology, the content areas for tabs are called "panes". The pane_selector selects the content areas that correspond to the individual tabs. For example, the selector: div.panes > div selects all div elements that are direct children of the div.panes element. The first pane is assigned to the first tab and second to the second tab and so on. This selector does not select any root element and it should return as many elements as you have tabs.
The value for this argument can also be a jQuery object that contains the required panes.
2nd argument: configuration
The second argument for the initialization call is the configuration object. It can be either a function in which case it will correspond to an onBeforeClick event listener or it can be a complex object with many different configuration options. Here is the full list of available options:
Note: If you want to instantiate multiple tabs with the same call then you should read this.
property | default | description |
---|---|---|
current | 'current' |
The CSS class name for the currently active tab. |
effect | 'default' |
The effect to be used when a tab is clicked. This can dramatically change the behaviour of the tabs. Here are the available built-in effects:
You can also make your own effects. |
event | 'click' |
Specifies the event when a tab is opened. By default, this happens when the user clicks on the tab. Other valid values are 'mouseover' and 'dblclick'. |
fadeInSpeed | 200 |
Since 1.0.1. Only available when used together with the "fade" effect. This property defines how fast (in milliseconds) the opened pane reveals its content. |
fadeOutSpeed | 0 |
Since 1.1.0. Only available when used together with the "fade" effect. This property defines how fast (in milliseconds) the opened pane hides its content. A positive value here will result in a "crossfade" effect which is demonstrated here. |
history | false |
Since 1.2.0. Enables support for browser's "back button" so that when a user clicks on the back or forward buttons the tabs are opened accordingly. Requires that the History tool is included on your page. |
initialIndex | 0 |
Specifies the tab that is initially opened when the page loads. This automatically triggers a click event for the tab specified in this property. Specifying null or a negative number here will not trigger the click event upon page load causing all tabs to be initially closed. |
rotate | false |
Since 1.1.0. When the last tab is open and the next() API call is invoked, then the tabs will start from the beginning and when the first tab is open and the prev() call is invoked the tabs will advance to the last tab. This is demonstrated in here. |
tabs | 'a' |
A selector for elements that are used as tabs inside the root element. If none are found then the direct children of the root element are used as tabs. |
Initializing multiple Tabs
Typically you have one Tabs instance on your page and your panes can be placed anywhere on the page. It is possible to instantiate multiple Tabs with the same call. For example, if you have multiple root elements with a CSS class name "tabs", they can all be instantiated like this:
$(".tabs").tabs(".panes <> div");
So how do we know which panes belong to which tabs? The solution is to enclose each tab/pane combination inside a wrapper element. For example:
<!-- 1st Tabs and associative panes inside a wrapping DIV element -->
<div>
<ul class="tabs">
...
</ul>
<div class="panes">
...
</div>
</div>
<!-- 2nd Tabs and accociative panes inside a wrapping DIV element -->
<div>
<ul class="tabs">
...
</ul>
<div class="panes">
...
</div>
</div>
Now all tabs will work individually. Note that instantiating Tabs with one call as in above example is different than doing multiple separate identical calls. Of course you can always instantiate your Tabs separately but you need to supply different selector for the panes. For example:
// two Tabs instantiation calls with different selectors
$("#tabs1").tabs("#panes1 <> div");
$("#tabs2").tabs("#panes2 <> div");
// done.
With accordion setups you typically have a common root element for both the tabs and the panes so there is no need for a separate wrapper element. You should take a look at the demo about Multiple Tabs and Accordion instances.
Events
Make sure you have read about Events in jQuery Tools. All event listeners receive the Event Object as the first argument.
event | When does it occur? |
---|---|
onBeforeClick | Before a tab is clicked. The second argument is the index number of the tab to be clicked. |
onClick | After a tab is clicked. The second argument is the index number of the tab being clicked. |
Scripting API
This tool has a useful API for scripters. A lot of effort has been put into it so that you can enrich the tabbing experience. You can get a handle to the API in numerous ways as described in the User's Manual. Here is an example of how to access the API:
// get handle to the api (must have been constructed before this call)
var api = $("ul.tabs").data("tabs");
// advance to the next tab
api.next();
// send google analytics when tabs are clicked
api.onClick(function(index) {
_tracker._trackEvent("Documentation", "Tabs", "tab " + index);
});
Here is a list of all API methods:
method | return value | description/example |
---|---|---|
click(index) | API |
Activates the tab specified in the argument. The argument can be either an integer number specifying the tab index (starting from 0) or, when the tabs are a tags, it can be the href attribute as a quoted string. |
destroy() | API |
Since 1.2.3 completely removes existing tabs instance. |
getConf() | Object |
Since 1.0.1. Returns the configuration object for the tabs instance. This object can be modified and the changes are dynamically reflected in the behaviour of the tabs. |
getCurrentPane() | jQuery |
Returns the current pane as a jQuery object. |
getCurrentTab() | jQuery |
Returns the current tab as a jQuery object. |
getIndex() | integer |
Returns the current tab index number. |
getPanes() | jQuery |
Returns the panes as a jQuery object. |
getTabs() | jQuery |
Returns the tabs as a jQuery object. |
next() | API |
Advances to the next tab. |
prev() | API |
Advances to the previous tab. |
Making custom effects
If you want to make custom effects you should use the $.tools.tabs.addEffect method. This method is "static", meaning that you don't have to have the tabs API (or instance) already loaded. You can add effects before any tabs are constructed.
This method accepts two arguments. The first argument is the effect name and the second argument is the function that performs the required functionality when a tab (or accordion header) is clicked. You can use this method to modify existing effects or add new ones. Here is an example:
// adds an effect called "myEffect" to tabs
$.tools.tabs.addEffect("myEffect", function(tabIndex, done) {
/* here you'll write your effect. the 'this' variable points to the API */
});
Your effect function accepts two arguments. The first, tabIndex, is the tab index number and the second, done, is a function that you must call after your effect finishes its job. This can be accomplished by calling done.call(); .
The default effect
Effects are actually quite easy to implement. The default effect, for example, does the following:
$.tools.tabs.addEffect("default", function(tabIndex, done) {
// hide all panes and show the one that is clicked
this.getPanes().hide().eq(tabIndex).show();
// the supplied callback must be called after the effect has finished its job
done.call();
});
Look in the tab's source code for more examples of the available effects. The basic idea is to use your jQuery skills together with the API methods. Here you can see a demo about a custom accordion effect.