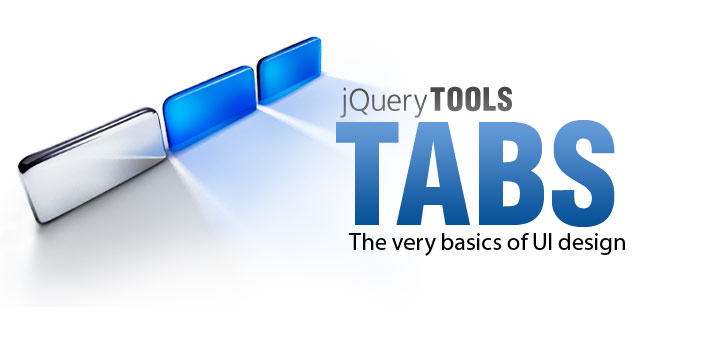
Making wizards with the tabs
The tabs can be easily used to make wizard-like interfaces. The following example uses next/prev buttons that use the tabs API to navigate between the panes. We also have a checkbox that must be checked if the user wants to advance to the next pane.
You can also see an exposing effect when you start using the wizard. This is an effective way to shift the user's focus to the task at hand.
An imaginary basket is here...
An imaginary order is here...
HTML coding
We have a div tag with its id equal to "wizard" as the root element, but otherwise the setup is similar to the minimal setup.
<!-- root element for the wizard -->
<div id="wizard">
<!-- tabs -->
<ul class="tabs">
<li><a href="#" class="w2">Personal info</a></li>
<li><a href="#" class="w2">Shopping basket</a></li>
<li><a href="#" class="w2">Review order</a></li>
</ul>
<!-- panes -->
<div class="panes">
<div> .. the form .. </div>
<div> .. the basket .. </div>
<div> .. the order .. </div>
</div>
</div>
Exposing effect
This JavaScript coding enables the mask effect with the custom background color: #789. The effect becomes active when the root element is clicked. Of course, this effect is optional and can be easily removed.
// get container for the wizard and initialize its exposing
var wizard = $("#wizard_tabs").expose({color: '#789', lazy: true});
// enable exposing on the wizard
wizard.click(function() {
$(this).expose().load();
});
The tabs
Here is the tabs setup. The complexity comes from the checkbox behaviour. We require the user to accept our "terms and conditions" before it is possible to move forward. The trick is to return false inside the onBeforeClick event.
// enable tabs that are contained within the wizard
$("ul.tabs", wizard).tabs("div.panes > div", function(event, index) {
/* now we are inside the onBeforeClick event */
// ensure that the "terms" checkbox is checked.
var terms = $("#terms");
if (index > 0 && !terms.get(0).checked) {
terms.parent().addClass("error");
// when false is returned, the user cannot advance to the next tab
return false;
}
// everything is ok. remove possible red highlight from the terms
terms.parent().removeClass("error");
});
Navigational buttons
Here is how the navigational buttons are setup. First we get a handle to the API and then we assign custom click events to the buttons that are contained inside the wizard.
// get handle to the tabs API
var api = $("ul.tabs", wizard).data("tabs");
// "next tab" button
$("button.next", wizard).click(function() {
api.next();
});
// "previous tab" button
$("button.prev", wizard).click(function() {
api.prev();
});