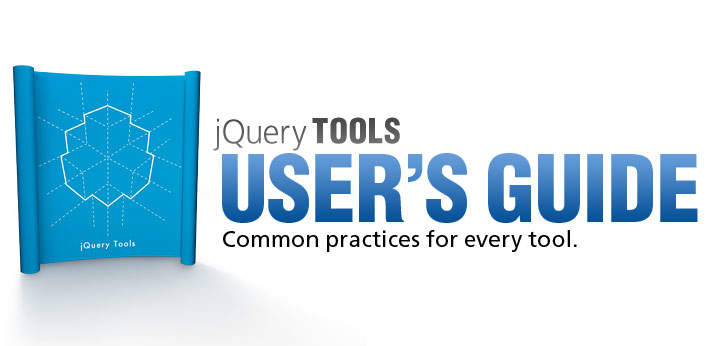
jQuery tools performance
This result has been identified by Yahoo engineers in their famous article Best Practices for Speeding Up Your Web Site. Most front-end time is tied up in downloading all the components on the page: images, stylesheets, scripts, Flash, etc. As a website developer, you should focus on this fact first rather than focusing on other areas such as backend optimization. Here are the primary rules that make your site work faster:
1. Make fewer HTTP requests
2. Use a Content Delivery Network (CDN)
3. Add an Expires header
4. GZIP components
5. Minify the JavaScript
By focusing on these areas, you can improve your website performance by roughly 70-80%. If this is hard to believe, I suggest you read the Yahoo white-paper.
jQuery Tools respects all 5 rules
By including jQuery Tools on your pages with following statement:
<script src="http://cdn.jquerytools.org/1.2.6/full/jquery.tools.min.js">
</script>
You should respect all five of the Yahoo performance rules mentioned above. We are offering this high performance content delivery network (CDN) for free. You can use it in all environments including production environments. Enjoy! If you are concerned about the file size you should download a combined script that contains only those tools that you really need and follow the principles that are mentioned in this document.
1. Make fewer HTTP requests
You might be familiar with following setup at the beginning of your page:
<script src="js/jquery.js"></script>
<script src="js/tabs.js"></script>
<script src="js/lightbox.js"></script>
<script src="js/another-plugin.js"></script>
And immediately after that setup you have noticed that your pages are much slower and you still have more plugins to be included. What an unbearable situation. This is the biggest reason for slow pages - lots of included scripts, CSS files and images. With jquery.tools you'll end up having the following setup:
<script src="http://cdn.jquerytools.org/1.2.6/full/jquery.tools.min.js">
</script>
This will include both the latest jQuery library and all the tools in a single file. Due to the fact that these tools are very generic in nature you may find yourself in a situation that you don't need a lot of other libraries on your pages. Many times you have your own site-wide JavaScript library that does all the custom stuff you need. If you are still in doubt that this is important, you should take a look at following online tests by Steve Souders:
The first example has five included scripts and it takes roughly 3 seconds to load and the second one has the same scripts in one combined file and it takes roughly 1 second to load - that is a noticeable improvement.
2. Use a CDN
CDN stands for Content Delivery Network and is a collection of web servers distributed across the globe to deliver content to users more efficiently. When a user accesses a file from Europe, then it is served from a server that is located in Europe. The same with with users in the United States and Asia.
Our CDN service is kindly provided by Max CDN and you can use it freely in any situation. Enjoy! Here is their global server distribution at the moment - they are all serving these tools quickly:
location | servers |
---|---|
North America | Chicago, Miami, Dallas, New York, Los Angeles, Atlanta, Seattle, Washington, DC/Ashburn, San Jose |
Europe | Amsterdam, Frankfurt and London in the UK |
Asia | Spring 2010 |
Generally, the files fetched from a CDN load 15-20% faster than without a CDN. You can prove this to yourself with this live example. If your website is viewed globally, we strongly recommend that you make a deal with a CDN provider. It is not a very complex procedure.
3. Add an Expires header
Adding an Expires header to your components with a date in the future makes them cacheable, reducing the load time of your pages. This won't affect performance the first time users hit your page, but on subsequent page views it could reduce response times by 50% or more. So we are talking about serious improvements here. For example, in Java Servlets you'll do this with the following call:
response.setHeader("Cache-Control", "max-age=315360000");
This will set the Expires header 10 years ahead which will do the job. The Tools under http://cdn.jquerytools.org have their Expires headers set correctly.
4. GZIP components
This is another huge performance saver and cuts down the file size roughly 65%. All modern browsers support gzipped files and are able to extract them before usage. There is absolutely no reason why you shouldn't compress them.
Approximately 90% of today's Internet traffic travels through browsers that claim to support gzip. You can test the response time improvement of gzipped components from this live example. The Tools under http://cdn.jquerytools.org are gzipped. Look at your web server documentation on how to enable gzipping. This is possible in every major server such as Apache, Tomcat or lighttpd.
5. Minify JavaScript
Minification is the practice of removing unnecessary characters from code to reduce its size thereby improving load times. When code is minified all comments are removed, as well as unneeded white space characters (space, newline, and tab). In a survey of ten top U.S. web sites, minification achieved a 21% size reduction.
jQuery Tools are minified with the Google Closure Compiler.
The Closure Compiler is a JavaScript minifier designed to be 100% safe and yield a higher compression-ratio than most other tools.
Note: Although packed scripts usually have smaller file sizes, they offer less performance than minified scripts. See the details of various compression schemes in this JavaScript Library Loading Speed article. For this reason, jQuery Tools are minified and not packed.