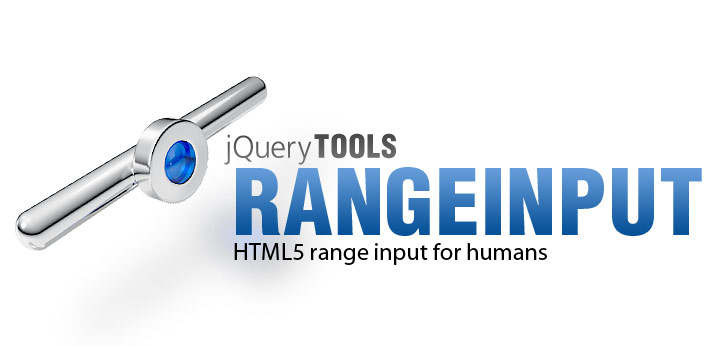
Rangeinput
This tool brings HTML5 range input to all browsers in such a way that you can make it look and behave like you want. This small candy weights only 2.2 Kb.
Change the value of the above range either by dragging the handle, clicking on the slider or changing the value of the input field. When you have the focus on the input field you can change the value with your Arrow Keys or with VIM keyboard shortcuts HJKL. PageUp and PageDown go 10 steps forward and backward.
HTML5 range input
This tool brings HTML5 <input type="range" /> support for all browsers in a consistent and standardized manner today. The range field is just like any other form field which can be scripted and submitted. Opera and Safari will render range input even if JavaScript is disabled.
For nerds and humans
Call $(":range").rangeinput(); and all your HTML5 range inputs are working. End users can pick values by dragging a slider or using the keyboard arrow keys. Hackers can use VIM keyboard shortcuts to move the range back and forth.
Styleable
You can design ranges to fit the overall feel of your site. Use images and even the latest CSS3 techniques if you like. Every bit of the range is skinnable. The default CSS class names can be changed and you can design both horizontal and vertical ranges. Professional example skins are provided and you may use them for free.
File size: 2.20 Kb!
It contains everything you need: configurability, skinning, orientation, standards based behaviour, scripting API and an event model. And as a bonus you'll get a generic drag utility! Last, but not least: a smaller codebase is easier to control and results in snappier behaviour.
Usage
Here is a quick preview of how this tool is used:
<!-- styling for the range -->
<link rel="stylesheet" type="text/css" href="range.css"/>
<!-- a couple of HTML5 range inputs with standard attributes -->
<input type="range" name="range1" min="50" max="500" step="20" value="100" />
<input type="range" name="range2" min="0" max="1500" step="50" value="450" />
<!-- select all range inputs and make them ranges -->
<script>
$(":range").rangeinput();
</script>
Now your cross-browser range inputs are available. The browser's built-in range input is replaced by a normal text input to keep it from messing things up. Essentially the original range inputs are replaced by new input fields where the type attribute has been changed to "text". For further details take a look at the demo: minimal setup for range input.
Demos
- Minimal setup for rangeinput
- A couple of vertical ranges
- Multiple small ranges
- A custom scrollbar for a DIV
Configuration
Property | Default | Description |
---|---|---|
css | Object |
CSS class names used by the generated range. Here are the
default values that you can change:
css: { |
keyboard | true | Whether keyboard shortcuts are enabled when the focus is on the input field. Both arrow keys and VIM shortcuts are supported. The page-up and page-down keys move the range by 10 steps. |
max | 100 | Maximum value. |
min | 0 | Minimum value. |
precision | 0 | By default the value's precision (the amount of decimal places) is determined by the value of the step configuration option. For example a value 0.01 results in a precision value 2 (two decimal places). You can override the automatic precision calculation with this configuration option. |
progress | false | Whether the progress bar is shown and its width is adjusted accordingly. |
speed | 200 | Animation speed for the drag handle when the range is clicked. |
step | 'any' |
A number that defines the size of a single step (the granularity of the value) limiting the amount of allowed values. A zero value or the string "any" disables stepping. The following range control only accepts values in the range from 0 to 1 and allows 100 steps within that range: <input name=opacity type=range min=0 max=1 step="0.01"> |
value | 0 | Initial value. |
vertical | false | If the slider element's height is larger than its width, the tool assumes that the range is vertical. You can override the assumption with this configuration variable. |
Input attributes
You can also configure the range by supplying valid HTML5 attributes for the range input field. For example:
<input type="range" value="40" min="20" max="400" step="20" />
Attribute | Description |
---|---|
disabled | Whether the range is completely disabled. Both the slider and the input field are disabled. The disabled attribute can be toggled dynamically by scripting. |
max | Maximum value. |
min | Minimum value. |
readonly | When enabled, the range is functional but the associative input field cannot be edited. |
step | The amount of steps the range takes between min and max. |
value | Initial value. |
Skinning
Once the range is initialized the following kind of HTML layout is constructed:
<div class="slider">
<div class="progress" style="width: 200px; display: block;"></div>
<a class="handle" style="left: 200px;"></a>
</div>
<input type="text" value="4" step="10" max="20" min="2" class="range">
There are two elements. The rangeinput and the input. Inside the rangeinput there is a progress bar and a drag handle. Here the handle has been dragged to position 200px, and the progress bar follows if it is enabled. The original range input is placed after the rangeinput component with its type attribute changed to "text". When it comes to skinning it's all about making your CSS against this structure. Here is one example skin which uses only one background gradient image.
Scripting API
First make sure you have familiarized yourself with jQuery Tools scripting.
Method | Return value | Description |
---|---|---|
getConf | Object |
Returns the configuration of the current instance. |
getHandle | jQuery |
Returns the drag handle element. |
getInput | jQuery |
Returns the input element. |
getProgress | jQuery |
Returns the progress element. The one that "follows" the handle. |
getValue | Number |
Returns the current value of the range. |
next | API |
Advance to the next step (value). |
prev | API |
Return to the previous step (value). |
setValue(value) | API |
Sets the range value to the one specified in the argument. |
step(amount) | API |
Changes the range value by the value given in the step configuration option multiplied by amount. The default amount is 1. A negative value decreases the value and a positive value increases it. |
stepDown(amount) | API |
Decreases the value with the amount of steps given in the argument. identical to step(-amount). |
stepUp(amount) | API |
Increases the value with the amount of steps given in the argument. identical to step(amount). |
DOM API
The official HTML5 range input defines a couple of DOM methods and attributes which are being enabled by this tool.
Method | Return value | Description |
---|---|---|
stepDown(amount) |
|
Decreases the value with the amount of steps given in the argument. identical to step(-amount) in the API. |
stepUp(amount) |
|
Increases the value with the amount of steps given in the argument. identical to step(amount) in the API. |
Events
First make sure you have read about Events in jQuery Tools. All event listeners receive the Event Object as the first argument. Here is an example:
// select all range inputs and assign event listeners to them
$(":range").change(function(event, value) {
console.info("value changed to", value);
});
Event | When does it occur? |
---|---|
onSlide | When sliding occurs. You can cancel the action by returning false or calling preventDefault() for the event object. Note that assigning this event listener is performance heavy and may affect the smoothness of the sliding experience. |
change | After the range value is changed. If you slide the range by clicking on the underlying slider this is the only event that is being triggered. Note that the name is the same as the jQuery's change method and you can bind the event directly with that function. For other events you must use the bind method. This leads to more fluent syntax as shown in the example above. |