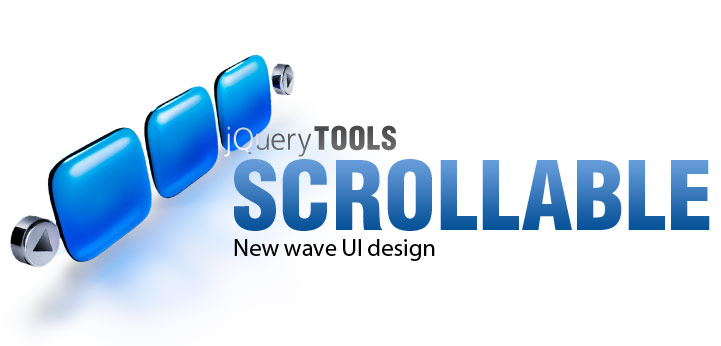
jQuery tools home page setup
This document describes how the jQuery Tools home page has been done. We recommend opening the standalone version of the demo in another browser window or tab while reading this document.
HTML coding
We have one root element div#scroll for everything and it also functions as the root element for the scrollable. The scrollable setup follows the same principles as the previous demo but this time we have two additions:
- An empty scrollable item which is shown when the page is loaded.
- An initial "intro page" which is shown on top of the empty scrollable item when the page is loaded
- the scrollable navigator (at the bottom of the items) also has an item for the first "empty" item but it is invisible making it impossible to click
<!-- root element for everything -->
<div id="scroll">
<!-- scrollable items -->
<div id="tools">
<!-- empty item -->
<div class="tool"> </div>
<!-- item #1: tabs -->
<div class="tool" style="background-image:url(tabs.jpg)">
... the content ...
</div>
<!-- .. rest of the items .. -->
<!-- the intro "page" -->
<div id="intro" class="tool" style="background:url(tools.jpg)">
... the content ...
</div>
</div>
<!-- thumbnails -->
<div id="thumbs" class="t">
<!-- intro page navigator button -->
<a id="t0" class="active"></a>
<!-- scrollable navigator root element -->
<div class="navi">
<!-- navigator item for the first empty "page" -->
<a style="display:none"></a>
<!-- navigator items for the rest of the scrollable items -->
<a id="t1"></a>
<a id="t2"></a>
<a id="t3"></a>
<a id="t4"></a>
<a id="t5"></a>
<a id="t6"></a>
</div>
</div>
</div>
CSS coding
As in every jQuery Tools setup, the CSS is the hardest part. We are not going very deep into the details on this. We follow the same principles on the scrollable elements as in the minimal setup (as always). The navigator items are done with a CSS sprite technique using this single background image. We define a different background position for each thumbnail and the position is changed when the item is being clicked, mouseovered or it is active. Here is the CSS setup for the first thumbnail.
#t0.active { background-position:-21px 0 !important; }
#t0:hover { background-position:-21px -180px; }
#t0:active { background-position:-21px -270px; }
JavaScript coding
The logic on the demo is that the first page is a special one and is outside the scrollable items. We use special logic for that. The scrollable itself is installed normally.
// initialize scrollable with navigator plugin
$("#scroll").scrollable({ items: '#tools'}).navigator();
Here is the special logic for the intro page.
// get handle to the scrollable api
var api = $("#scroll").data("scrollable");
// this callback does the special handling of our "intro page"
api.onStart(function(e, i) {
// when on the first item: hide the intro
if (i) {
$("#intro").fadeOut("slow");
// otherwise show the intro
} else {
$("#intro").fadeIn(1000);
}
// toggle activity for the intro thumbnail
$("#t0").toggleClass("active", i == 0);
});
// a dedicated click event for the intro thumbnail
$("#t0").click(function() {
// seek to the beginning (the hidden first item)
$("#scroll").scrollable().begin();
});
Note: the demo can also be navigated by using the arrow keys.