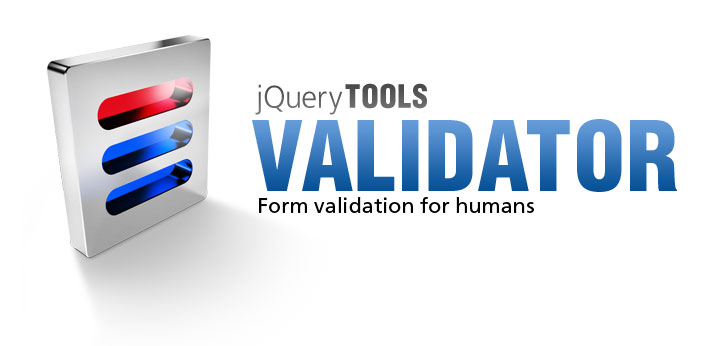
Validator events in action
In addition to the effect framework you can extend the validator with your own functions that are called when validation related events occur.
Here comes the validator events
The red border effect
Here is an example callback function that does the red border effect.
$("#myform").bind("onFail", function(e, errors) {
// we are only doing stuff when the form is submitted
if (e.originalEvent.type == 'submit') {
// loop through Error objects and add the border color
$.each(errors, function() {
var input = this.input;
input.css({borderColor: 'red'}).focus(function() {
input.css({borderColor: '#444'});
});
});
}
});
Displaying errors on the "wall"
Here we show the various ways to assigning callback functions for validator. It is not meant to be simple. It's meant to show you the different possibilities. All these callback functions print messages to a DIV with id "info".
// get handle to the info box
var info = $("#info");
// initialize validator and supply the onBeforeValidate event in configuration
$("#myform").validator({
onBeforeValidate: function(e, els) {
info.empty();
showEvent("onBeforeValidate", els);
}
// use jQuery's bind method
}).bind("onBeforeFail", function(e, els) {
showEvent("onBeforeFail", els);
// another listener with bind
}).bind("onFail", function(e, els) {
showEvent("onFail", els.length + " inputs");
});
// get handle to the Validator API
var api = $("#myform").data("validator");
// use API to assign an event listener
api.onSuccess(function(e, els) {
showEvent("onSuccess", els);
// we don't want to submit the form. just show events.
return false;
});
// event handler just for a single field
$(":email").oninvalid(function(e, message) {
showEvent("oninvalid", $(this));
});
// this function is used to show error on the info box
window.showEvent = function(eventName, input) {
var inputName = input.jquery ? input.attr("name") : input;
info.append("<p><strong>" + eventName + "</strong>: " + inputName + "</p>").fadeIn();
};