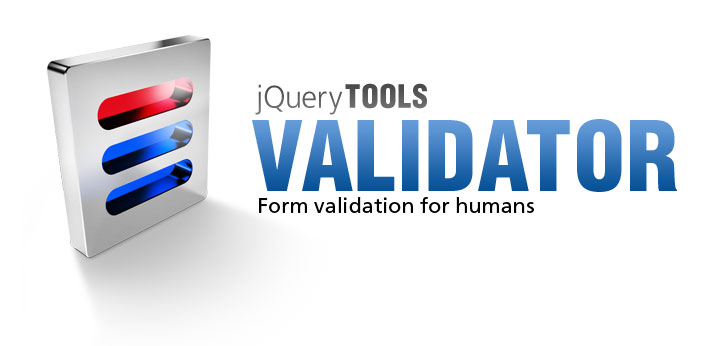
Error Summary
If you want alternate ways of displaying errors you can write a reusable effect for that.
Please fix these first
HTML coding
This effect contains a single error container for all validation errors. Here it is
<div id="errors">
<h2>Please fix these first</h2>
</div>
Styling the error container
The form itself uses this external stylesheet. We have added addional styling for the error message container. Nothing special here.
/* error container */
#errors {
background-color:#163356;
color:#fff;
width:400px;
padding:20px;
margin:5px auto;
display:none;
-moz-border-radius:5px;
-webkit-border-radius:5px;
}
/* title */
#errors h2 {
margin:-5px 0;
color:yellow;
}
The custom effect
Here is the custom effect. It is added with $.tools.validator.addEffect method as described in Making custom effects section of the validator documentation. The effect takes the container, makes it visible and adds the messages inside it. It is only triggered upon form submission and not when fields are edited.
// adds an effect called "wall" to the validator
$.tools.validator.addEffect("wall", function(errors, event) {
// get the message wall
var wall = $(this.getConf().container).fadeIn();
// remove all existing messages
wall.find("p").remove();
// add new ones
$.each(errors, function(index, error) {
wall.append(
"<p><strong>" +error.input.attr("name")+ "</strong> " +error.messages[0]+ "</p>"
);
});
// the effect does nothing when all inputs are valid
}, function(inputs) {
});
You need to place the script block after the HTML code or you can alternatively use jQuery's $(document).ready to execute the script as soon as the webpage's Document Object Model (DOM) has been loaded. Read more about that from the User's Manual.
Using the effect
Now we initialize the validator and also make a custom form submission logic.
// initialize validator with the new effect
$("#myform").validator({
effect: 'wall',
container: '#errors',
// do not validate inputs when they are edited
errorInputEvent: null
// custom form submission logic
}).submit(function(e) {
// when data is valid
if (!e.isDefaultPrevented()) {
// tell user that everything is OK
$("#errors").html("<h2>All good</h2>");
// prevent the form data being submitted to the server
e.preventDefault();
}
});