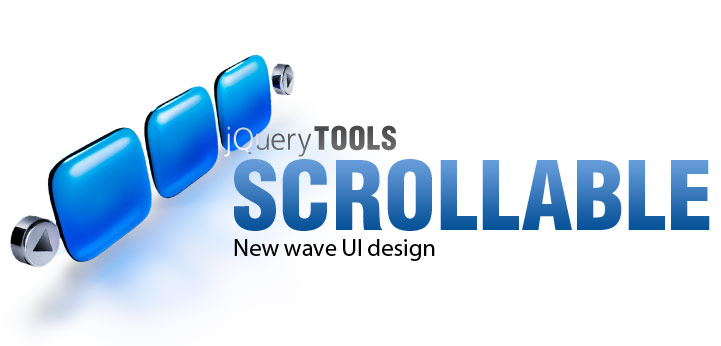
A scrollable registration wizard
Here is a registration wizard with primitive input validation logic and a "twitter" style message box for validation errors:
HTML coding
The HTML structure follows the same principles as the minimal setup for Scrollable with the addition of two special elements: #drawer for showing the error messages in "twitter style" and #status for displaying the page the user is currently on.
<!-- twitter style "drawer" for displaying validation errors -->
<div id="drawer">[ERROR MESSAGE]</div>
<!-- the form -->
<form action="#">
<!-- scrollable root element -->
<div id="wizard">
<!-- status bar -->
<ul id="status">
<li class="active"><strong>1.</strong> Create Account</li>
<li><strong>2.</strong> Contact Information</li>
<li><strong>3.</strong> Finalize</li>
</ul>
<!-- scrollable items -->
<div class="items">
<!-- pages -->
<div class="page">[ Any HTML content ]</div>
<div class="page">[ Any HTML content ]</div>
<div class="page">[ Any HTML content ]</div>
</div>
</div>
</form>
CSS coding
The CSS coding is the hardest part of this demo. Here are the most important parts taken from the scrollable-wizard.css which is used for styling the entire demo.
/* the root element for scrollable */
#wizard {
overflow:hidden;
position:relative;
}
/* scrollable items */
#wizard .items {
width:20000em;
clear:both;
position:absolute;
}
/* single scrollable item called ".page" in this setup */
#wizard .page {
padding:20px 30px;
width:500px;
float:left;
}
/* validation error message bar. positioned on the top edge */
#drawer {
overflow:visible;
position:fixed;
left:0;
top:0;
Scrollable setup
This is the easy part (again). Self explanatory.
var root = $("#wizard").scrollable();
Input validation logic
The wizard contains a simple validation logic that only checks that the required fields are not blank. It checks for input fields that are nested inside an li.required element and makes sure that they have a value. White space characters are not accepted. If you want to implement a proper validation logic please use the Validator Tool.
I have added lots of comments so that you'll understand what's happening. Notice that the "drawer" logic is a very simple one-liner although the effect is very useful.
// some variables that we need
var api = root.scrollable(), drawer = $("#drawer");
// validation logic is done inside the onBeforeSeek callback
api.onBeforeSeek(function(event, i) {
// we are going 1 step backwards so no need for validation
if (api.getIndex() < i) {
// 1. get current page
var page = root.find(".page").eq(api.getIndex()),
// 2. .. and all required fields inside the page
inputs = page.find(".required :input").removeClass("error"),
// 3. .. which are empty
empty = inputs.filter(function() {
return $(this).val().replace(/\s*/g, '') == '';
});
// if there are empty fields, then
if (empty.length) {
// slide down the drawer
drawer.slideDown(function() {
// colored flash effect
drawer.css("backgroundColor", "#229");
setTimeout(function() { drawer.css("backgroundColor", "#fff"); }, 1000);
});
// add a CSS class name "error" for empty & required fields
empty.addClass("error");
// cancel seeking of the scrollable by returning false
return false;
// everything is good
} else {
// hide the drawer
drawer.slideUp();
}
}
// update status bar
$("#status li").removeClass("active").eq(i).addClass("active");
});
The final tweak
We still need to make one optimization for the whole setup. If the user advances through those form fields with the TAB key we must make sure that the form page is validated before advancing to the next tab. This can be achieved with the following JavaScript snippet:
// if tab is pressed on the next button seek to next page
root.find("button.next").keydown(function(e) {
if (e.keyCode == 9) {
// seeks to next tab by executing our validation routine
api.next();
e.preventDefault();
}
});