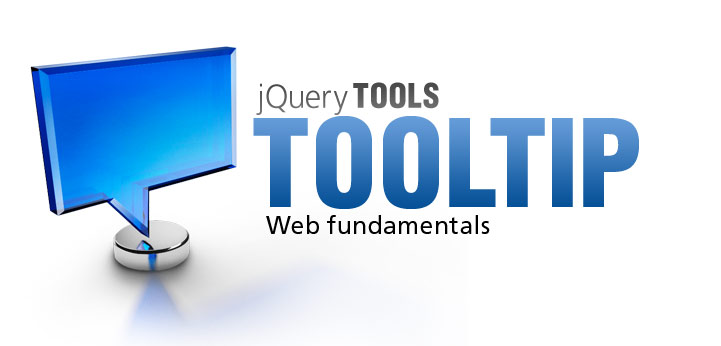
Using tooltips in form fields
Here you can see a tooltip when you put your focus on any of the following form fields. You can move between fields with your keyboard (using the TAB key) or with your mouse.
HTML coding
Here is our HTML structure. Each tooltip is specified in the title attribute of each form element:
<form id="myform" action="#">
<h3>Registration Form</h3>
<div id="inputs">
<!-- username -->
<label for="username">Username</label>
<input id="username" title="Must be at least 8 characters."/>
<br />
<!-- password -->
<label for="password">Password</label>
<input id="password" type="password" title="Make it hard to guess." />
<br />
<!-- email -->
<label for="email">Email</label>
<input id="email" title="We won't send you any marketing material." />
<br />
<!-- message -->
<label for="body">Message</label>
<textarea id="body" title="What's on your mind?"></textarea>
<br />
<!-- message -->
<label for="where">Select one</label>
<select id="where" title="Select one of these options">
<option>-- first option --</option>
<option>-- second option --</option>
<option>-- third option --</option>
</select>
<br />
</div>
<!-- email -->
<label>
I accept the terms and conditions
<input type="checkbox" id="check" title="Required to proceed" />
</label>
<p>
<button type="button" title="This button won't do anything">
Proceed
</button>
</p>
</form>
This form has minimal CSS styling and you can see it by looking at the standalone page's source code.
CSS coding
Here is our tooltip "skin". Everything is pure CSS without any images or background images:
/* simple css-based tooltip */
.tooltip {
background-color:#000;
border:1px solid #fff;
padding:10px 15px;
width:200px;
display:none;
color:#fff;
text-align:left;
font-size:12px;
/* outline radius for mozilla/firefox only */
-moz-box-shadow:0 0 10px #000;
-webkit-box-shadow:0 0 10px #000;
}
JavaScript coding
All tooltips are enabled with the following configuration. If you want to customize the events when the tooltip is shown, then you should read about event management from the tooltip documentation.
// select all desired input fields and attach tooltips to them
$("#myform :input").tooltip({
// place tooltip on the right edge
position: "center right",
// a little tweaking of the position
offset: [-2, 10],
// use the built-in fadeIn/fadeOut effect
effect: "fade",
// custom opacity setting
opacity: 0.7
});