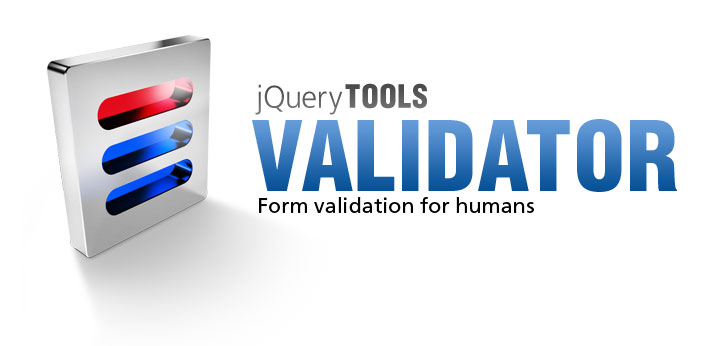
Minimal setup for validator
Here we have a form that has valid HTML5 syntax. We have used email, url and number inputs as well as a normal text input with a pattern attribute. The requred attribute is used to indicate a mandatory field. The old school but handy maxlength attribute makes it impossible to enter more than 30 characters.
HTML coding
<form id="myform">
<fieldset>
<h3>Sample registration form</h3>
<p> Enter bad values and then press the submit button. </p>
<p>
<label>email *</label>
<input type="email" name="email" required="required" />
</p>
<p>
<label>website *</label>
<input type="url" name="url" required="required" />
</p>
<p>
<label>name *</label>
<input type="text" name="name" pattern="[a-zA-Z ]{5,}" maxlength="30" />
</p>
<p>
<label>age</label>
<input type="number" name="age" size="4" min="5" max="50" />
</p>
<p id="terms">
<label>I accept the terms</label>
<input type="checkbox" required="required" />
</p>
<button type="submit">Submit form</button>
<button type="reset">Reset</button>
</fieldset>
</form>
JavaScript coding
This is the simple part. Simply initialize validator and you'll get all the HTML5 niceties.
$("#myform").validator();
You need to place the script block after the HTML code or you can alternatively use jQuery's $(document).ready to execute the script as soon as the webpage's Document Object Model (DOM) has been loaded. Read more about that from the User's Manual.
CSS coding
We have used one external stylesheet for the design. It has styling for the whole form. Here we focus on the validation and the error message:
/* error message */
.error {
/* supply height to ensure consistent positioning for every browser */
height:15px;
background-color:#FFFE36;
border:1px solid #E1E16D;
font-size:11px;
color:#000;
padding:3px 10px;
margin-left:-2px;
/* CSS3 spicing for mozilla and webkit */
-moz-border-radius:4px;
-webkit-border-radius:4px;
-moz-border-radius-bottomleft:0;
-moz-border-radius-topleft:0;
-webkit-border-bottom-left-radius:0;
-webkit-border-top-left-radius:0;
-moz-box-shadow:0 0 6px #ddd;
-webkit-box-shadow:0 0 6px #ddd;
}