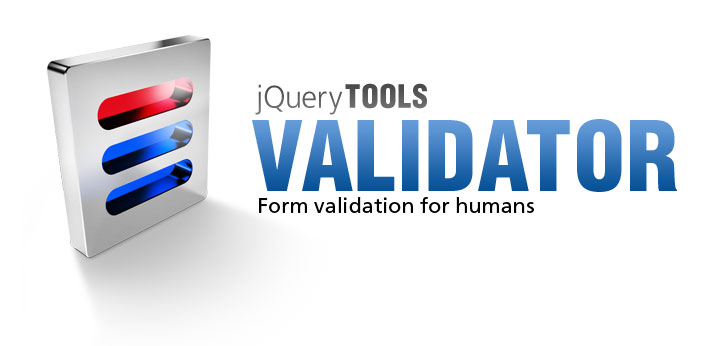
Custom input types and attributes
Here we make a custom time input with custom minlength and data-equals attributes. New wave form development. jQuery Tools Validator makes it easy.
HTML coding
Here it is. A HTML5 form together with our new attributes:
<form id="myform" class="cols">
<h3>HTML5 form with custom inputs</h3>
<fieldset>
<label> email * <input type="email" required="required" minlength="9" /> </label>
<label> username * <input type="text" name="username" minlength="5" /> </label>
<label> Password <input type="password" name="password" minlength="4" /> </label>
<label> Password check <input type="password" name="check" data-equals="password" /> </label>
</fieldset>
<fieldset>
<label> website * <input type="url" required="required" /> </label>
<label> name * <input type="text" name="name" required="required" maxlength="30" /> </label>
<label> age <input type="number" name="age" size="4" maxlength="3" /> </label>
<label> time <input type="time" name="time" maxlength="8" /> </label>
</fieldset>
<div class="clear"></div>
<button type="submit">Submit form</button>
</form>
Custom validators
Implementing new attributes and input type is very easy with this tool. So easy that you'll end up developing new ones regularly. Each new attribute and input type requires a new custom validator function. They are added to the Validator tool with the $.tools.validator.fn method.
time input type
Time input belongs to the HTML5 standard. Here we implement it. A valid input has two numbers followed by ":" followed by two numbers. For example: 12:44.
// Regular Expression to test whether the value is valid
$.tools.validator.fn("[type=time]", "Please supply a valid time", function(input, value) {
return /^\d\d:\d\d$/.test(value);
});
This is the most common way of defining a custom validator. The first argument matches all inputs you want to validate. Here we select input fields whose type attribute is equal to "time". The second argument is the error message that is shown when validation fails. The third argument is the actual validator function; it must return true if the value is valid. The value is supplied as the second argument to the validator function.
data-equals attribute
Our next validator ensures that one field's value is equal to another field's value. For example data-equals="password" means that the value must equal to a field named "password". The data- prefix makes it valid HTML5.
$.tools.validator.fn("[data-equals]", "Value not equal with the $1 field", function(input) {
var name = input.attr("data-equals"),
field = this.getInputs().filter("[name=" + name + "]");
return input.val() == field.val() ? true : [name];
});
Here you can see the backreference $1 in the error message. It is being replaced by the return value of the validator function when a validation fails. You can have zero or more backreferences and they must be contained in an Array. Also note that inside the validator this is a reference to the validator API.
minlength attribute
Here we make use of the non-standard minlength attribute. When making custom attributes you can use any attribute name you like and things will work. The downside is that custom names don't pass HTML validation but in an ideal world this shouldn't be the case.
$.tools.validator.fn("[minlength]", function(input, value) {
var min = input.attr("minlength");
return value.length >= min ? true : {
en: "Please provide at least " +min+ " character" + (min > 1 ? "s" : ""),
fi: "Kentän minimipituus on " +min+ " merkkiä"
};
});
This time we return the error message and don't provide it as the second argument. This gives you the possibility to dynamically construct the message. You can return a simple string in which case it is assumed to be in English or you can return an object just as we do here.
Localization
Here we localize our time input with the $.tools.validator.localizeFn call.
$.tools.validator.localizeFn("[type=time]", {
en: 'Please supply a valid time',
fi: 'Virheellinen aika'
});
And here are all the messages contained inside the validator as seen from the Firebug console.
You can read more about custom validators and localization from the validator documentation.
Validator initialization
Once the custom validators have been defined we initialize validation for our form. We tweak the position of the error message with the offset configuration variable:
$("#myform").validator({
position: 'top left',
offset: [-12, 0],
message: '<div><em/></div>' // em element is the arrow
});
You need to place the script block after the HTML code or you can alternatively use jQuery's $(document).ready to execute the script as soon as the webpage's Document Object Model (DOM) has been loaded. Read more about that from the User's Manual.
CSS coding
We use two stylesheets for the job:
<!-- same styling as in minimal setup -->
<link rel="stylesheet" type="text/css"
href="/media/css/validator/form.css"/>
<!-- override it to have a columned layout -->
<link rel="stylesheet" type="text/css"
href="/media/css/validator/columns.css"/>
Pure CSS arrow
One interesting feature in columns.css is the arrow that is done with CSS only. No images. Here is the trick:
/* pure CSS arrow */
.error em {
display:block;
width:0;
height:0;
border:10px solid;
border-color:#FFFE36 transparent transparent;
/* positioning */
position:absolute;
bottom:-17px;
left:60px;
}